The Basics of Conversations
Google has portrayed “conversations” as being a major thing in Android 11. And, in truth, it is one of the few user-facing features of Android 11. However, from a programming standpoint, “conversations” may be just a minor extension to what you are already doing with notifications.
Conversation Presentation
Conversation notifications are placed above regular notifications in the notification shade:
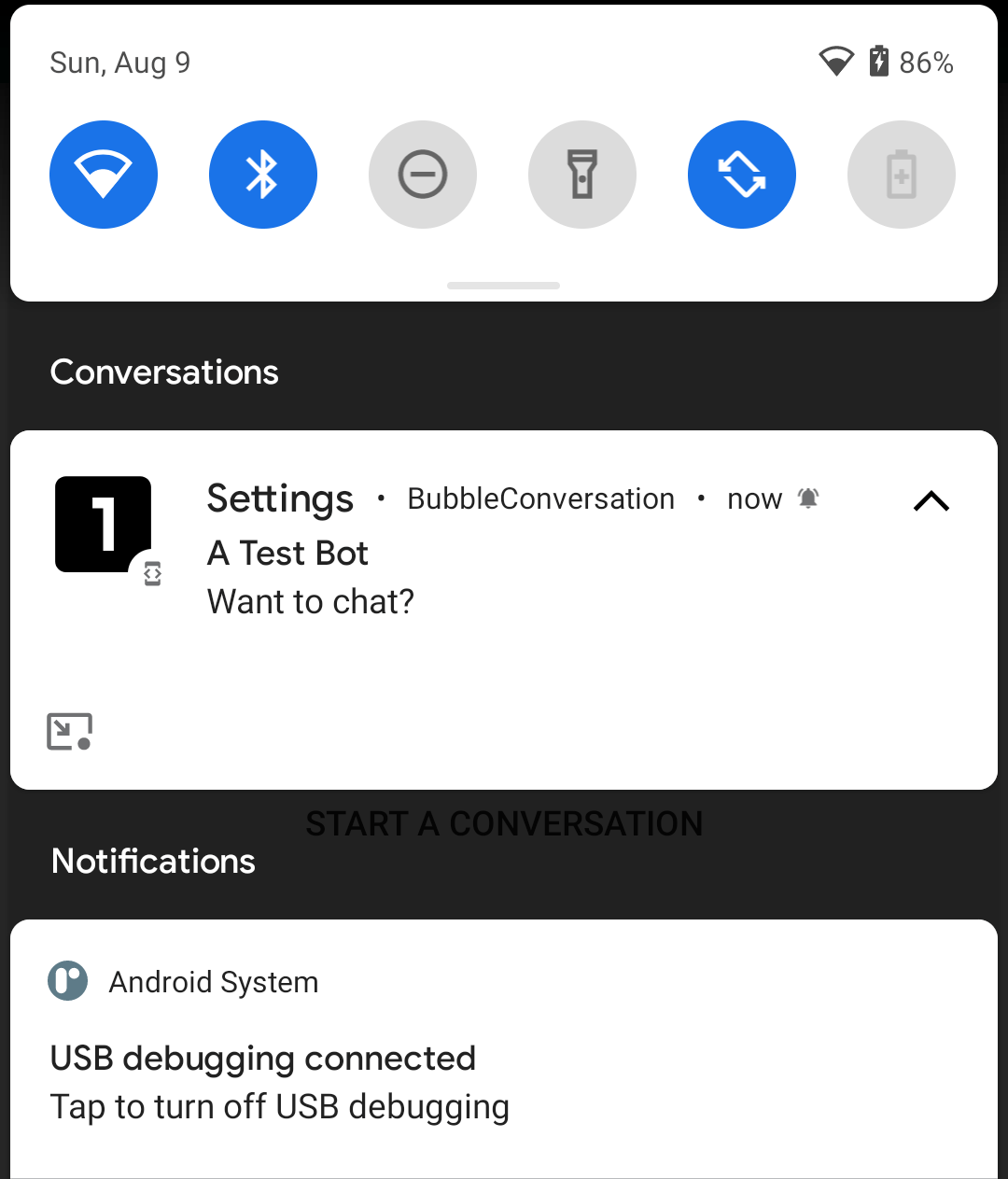
They have slightly different presentation, with a greater emphasis on a developer-supplied icon, typically representing the person (or bot or other non-corporeal entity) with which the “conversation” is being held. Tapping the caret toggles between expanded and collapsed perspectives, and long-pressing the caret can bring up options, such as making the conversation be priority or silent:
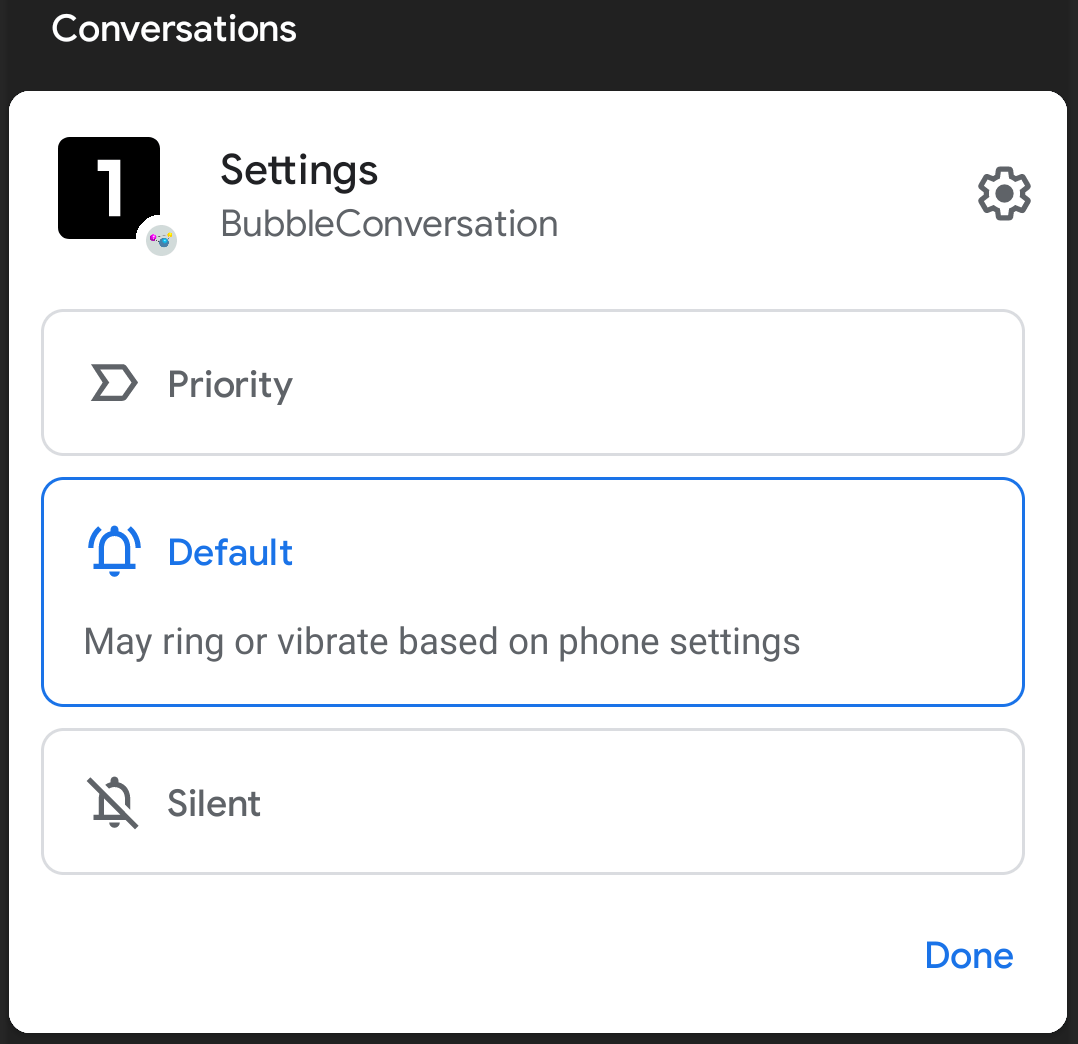
But, mostly, this is just a MessagingStyle
notification.
However, you may notice an icon in the lower-left of the notification. If this is available, the user can turn this conversation into a bubble by tapping on it. This implies that you have set up this notification with the metadata needed for bubbles, and we will explore that and the rest of the bubble setup and presentation later in the chapter.
Constructing a Conversation
A conversation notification is a MessagingStyle
notification with a “long-lived” shortcut associated with it. The documentation states that the shortcut must be associated with Person
objects in the conversation, though this does not appear to be required. The shortcut that gets created not only helps set up your conversation, but it appears as a real shortcut, such as on a long-press of your launcher icon. As such, the shortcut needs to be real and to work, even though the Intent
associated with that shortcut is not used with the notification itself.
The BubbleConversation
sample module in the book’s sample project shows a basic recipe for getting a bubble to display and work, in the context of a “conversation”-style notification. It has an activity with a really big button that, when clicked, raises a conversation-style notification.
This code sets up a NotificationCompat.Builder
for a conversation:
val shortcutInfo = ShortcutInfoCompat.Builder(this, SHORTCUT_ID)
.setLongLived(true)
.setShortLabel("Settings")
.setIntent(Intent(Settings.ACTION_SETTINGS))
.setIcon(IconCompat.createWithResource(this, R.drawable.ic_one))
.build()
ShortcutManagerCompat.pushDynamicShortcut(this, shortcutInfo)
val builder = NotificationCompat.Builder(
appContext,
CHANNEL_WHATEVER
)
.setSmallIcon(R.drawable.ic_notification)
.setContentTitle("Um, hi!")
.setBubbleMetadata(bubble)
.setShortcutInfo(shortcutInfo)
val person = Person.Builder()
.setBot(true)
.setName("A Test Bot")
.setImportant(true)
.build()
val style = NotificationCompat.MessagingStyle(person)
.setConversationTitle("A Fake Chat")
style.addMessage("Want to chat?", System.currentTimeMillis(), person)
builder.setStyle(style)
Most of this code is mostly there to set up the MessagingStyle
notification. And the setBubbleMetadata()
call is tied to bubbles, as we will see later in the chapter.
To make this be categorized as a conversation, we:
- Create a
ShortcutInfoCompat
usingShortcutInfoCompat.Builder
, providing enough information to create valid shortcut, plussetLongLived(true)
- Register that shortcut with
ShortcutManagerCompat
, in this case viapushDynamicShortcut()
- Attach that shortcut to the notification using
setShortcutInfo()
onNotificationCompat.Builder
In this case, the shortcut itself just launches the device’s Settings app. A more typical solution would be to have it launch something related to the conversation or its participants.
Note that the icon used as the primary visual indicator in the conversation is the icon associated with the shortcut (in this case, R.drawable.ic_one
). Hence, you will want to set that icon to be something that represents the conversation or its participants, further emphasizing the need for the shortcut itself to be tied to the same things.
Prev Table of Contents Next
This book is licensed under the Creative Commons Attribution-ShareAlike 4.0 International license.