Our Initial Resource Types
Our starter app contains six types of resources, though two of them (drawables and mipmaps) are pretty much the same thing.
Layouts
The resource type that will consume most of your time is the layout resource. This describes a chunk of our app’s user interface. That chunk could be:
- A screen
- A row in a list
- A cell in a grid
- A reusable piece that you want to apply to several different screens
- And so on
Layout resources are XML files that either you create by hand or create through the use of drag-and-drop GUI builders built into Android Studio. We will be spending quite a bit of time covering layout resources throughout this book, starting with a chapter on widgets, our smallest pieces of a layout resource.
The starter project has a fairly simple layout… though it could be even simpler:
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
As we will see, XML elements generally will map to widgets (things that users touch) and containers (things that organize widgets and other containers). So, here, we have a ConstraintLayout
container that wraps around a single TextView
widget.
In the chapter on widgets, we will explore this XML structure in detail, plus show you how you can set up this XML through the drag-and-drop GUI builder.
Drawables and Mipmaps
All apps have some amount of artwork, mostly in the form of icons. For example, most apps have an icon that will appear in the home screen or launcher app, that allows the user to bring up the app’s UI. Apps might have other icons in that UI, to appear on buttons or other tappable things. Some apps may use a “splash screen” as an introductory bit of UI, and so they have some large graphic that they want to use for that screen. And there are many other uses of artwork within an Android app, of relevance to some apps but perhaps not to others.
Sometimes, these graphics are downloaded from the Internet as part of running the app. Most of the rest are packaged with the app itself: the graphic designer creates the artwork and the developer arranges to use it in the app in the appropriate place.
Most of these pre-packaged bits of artwork are in the form of drawable and mipmap resources.
Many of these are bitmap images: PNG, JPEG, etc. They can also be:
- Vector art, imported from SVG files that your graphic designer might prepare in tools like Adobe Illustrator
- Specialized XML files, usually with rules for how to combine two or more other resources together
There are really boring technical distinctions between drawables and mipmaps, and tedious historical explanations for why we have two different resource types for the same stuff. For the purposes of this book — and so you do not fall asleep while reading it — you do not need to worry about all of that. The rules for the vast majority of Android developers are fairly simple:
- Your home screen launcher icon is a
mipmap
- Everything else is a
drawable
We will start exploring these resources more in an upcoming chapter where we change your launcher icon.
Strings
Keeping your labels and other bits of text outside the main source code of your application is generally considered to be a very good idea. In particular, it helps with internationalization (I18N) and localization (L10N). Even if you are not going to translate your strings to other languages, it is easier to make corrections if all the strings are in one spot instead of scattered throughout your source code.
Strings are one of the “values” resource types. So, in the values/
directory, we can have one or several files that contain string resources. Typically, you have just one such file, named strings.xml
.
The starter app’s strings.xml
file contains… not very much:
<resources>
<string name="app_name">Hello World</string>
</resources>
All files in the values/
directory will be XML files with a root <resources>
element. What appears inside that root element defines the actual resources contained in that file.
Inside strings.xml
, the <resources>
element contains just one child element: a <string>
, defining a single string resource. Each string resource has a name
, which is how we will refer to that string from elsewhere in the app. And, each string resource has a value, consisting of the text between the <string>
and </string>
tags. Here, we define app_name
to be “HelloWorld”.
The starter app does not have translations of this resource, but it could. For example, it could contain a res/values-es/
directory, containing strings to be used for devices whose locale is set to Spanish. In there, app_name
might be defined as “Hola Mundo”. On the fly, Android will choose the right translation to use, based on the translations that you provide and the locale of the device.
We will be working with a bunch of string resources in this book, and we will explore the issues of translations a bit more in a later chapter.
Colors
Another type of “values” resource is the color resource. As you might expect, it provides a symbolic name for colors. This allows us to give names that have semantic meaning (e.g., “the standard accent color”) and use those names in our code. It also then gives us one place to define what the actual color is for that name, so if we need to change the color, we can change it in one place.
Color resources are defined by <color>
elements in a “values” resource file. Convention says that your colors go into a colors.xml
resource file, and that is what the starter app has:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<color name="purple_200">#FFBB86FC</color>
<color name="purple_500">#FF6200EE</color>
<color name="purple_700">#FF3700B3</color>
<color name="teal_200">#FF03DAC5</color>
<color name="teal_700">#FF018786</color>
<color name="black">#FF000000</color>
<color name="white">#FFFFFFFF</color>
</resources>
As with our app_name
string resource, just having these colors does not cause anything to use those colors. That requires additional code or additional resources, ones that happen to reference these resources.
Styles and Themes
A place where color resources are often used is in style resources. Style resources are reminiscent of CSS stylesheets in Web development. Styles allow you to give a name to a collection of UI properties, then apply those properties to various scenarios.
One such scenario is where a style is used as a “theme”. This provides the defaults for UI properties for an entire activity, or perhaps even the entire app. The sample project defines one such theme, AppTheme
, in its themes.xml
file:
<resources xmlns:tools="http://schemas.android.com/tools">
<!-- Base application theme. -->
<style name="Theme.HelloWorld" parent="Theme.MaterialComponents.DayNight.DarkActionBar">
<!-- Primary brand color. -->
<item name="colorPrimary">@color/purple_500</item>
<item name="colorPrimaryVariant">@color/purple_700</item>
<item name="colorOnPrimary">@color/white</item>
<!-- Secondary brand color. -->
<item name="colorSecondary">@color/teal_200</item>
<item name="colorSecondaryVariant">@color/teal_700</item>
<item name="colorOnSecondary">@color/black</item>
<!-- Status bar color. -->
<item name="android:statusBarColor" tools:targetApi="l">
?attr/colorPrimaryVariant
</item>
<!-- Customize your theme here. -->
</style>
</resources>
The parent
attribute on the <style>
indicates that we are inheriting existing UI property definitions from something called Theme.MaterialComponents.DayNight.DarkActionBar
. That name has lots of pieces:
-
Theme
indicates that we are starting from the base system theme -
MaterialComponents
indicates that this theme comes from a library referred to as the Material Components for Android -
DayNight
indicates that the general look is dark text on a light background during the daytime, and light text on a dark background at night -
DarkActionBar
says that the “app bar” — the toolbar structure towards the top of the screen of most Android activities, formerly called an “action bar” — should have white icons on a dark background
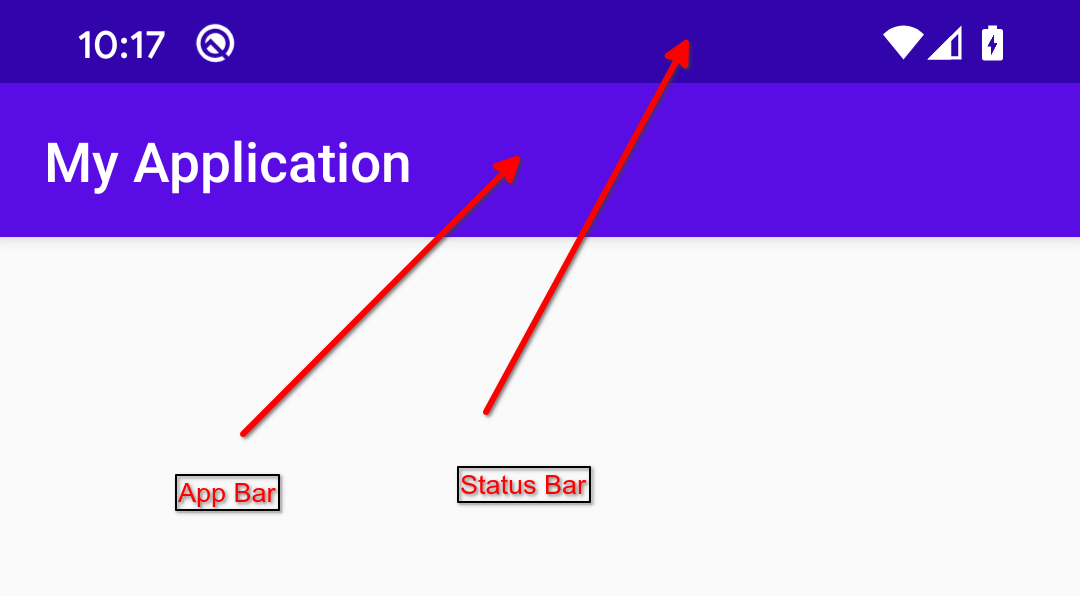
AppTheme
inherits from Theme.MaterialComponents.DayNight.DarkActionBar
, so we get lots of stuff “for free” as a result. We then override additional UI properties as we see fit, such as colorPrimary
, which the Material Components will use for the app bar background, the foreground text in the main UI area, and a few other roles.
The AppTheme
style refers to the color resources that were defined in the colors.xml
file. In resources, when you need to refer to another resource, you do so using the syntax @type/name
, where type
is the type of the resource (color
, string
, drawable
, mipmap
, etc.), and name
is the name of the resource. For “values” resources, like our colors, the name comes from the name
attribute of the element that defines the resource. For all other types of resources, the name comes from the filename of the resource file, without the file extension. So, here, @color/colorPrimary
refers to the colorPrimary
color resource.
Note that the resource references do not include any of those suffixes on the directory names that we use for resource sets. If you look in the various directories for mipmap resources, you will see that we have six different variations of an ic_launcher
mipmap:
mipmap-anydpi-v26/ic_launcher.xml
mipmap-hdpi/ic_launcher.png
mipmap-mdpi/ic_launcher.png
mipmap-xhdpi/ic_launcher.png
mipmap-xxhdpi/ic_launcher.png
mipmap-xxxhdpi/ic_launcher.png
However, when things like our manifest refer to these, it is always as @mipmap/ic_launcher
. Android will decide, on the fly, which of these six definitions to use, based on the rules encoded in those directory names and the configuration of the device at the time we are trying to use the resource. We will get much more into all of that complexity later in the book.
Prev Table of Contents Next
This book is licensed under the Creative Commons Attribution-ShareAlike 4.0 International license.