SeekBar
Sometimes, you want the user to pick a number along some range, such as a percentage from 0% to 100%. You could use an EditText
and then have data validation to handle illegal entries (e.g., numbers outside of your desired range).
Or, you can use a SeekBar
.
SeekBar
allows the user to slide a “thumb” along a bar, where different thumb positions represent different values along a range from 0 to a maximum value that you specify (the default maximum is 100).
The bottom of our layout is a SeekBar
:
<SeekBar
android:id="@+id/seekbar"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginTop="@dimen/margin_row"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@id/radioGroup" />
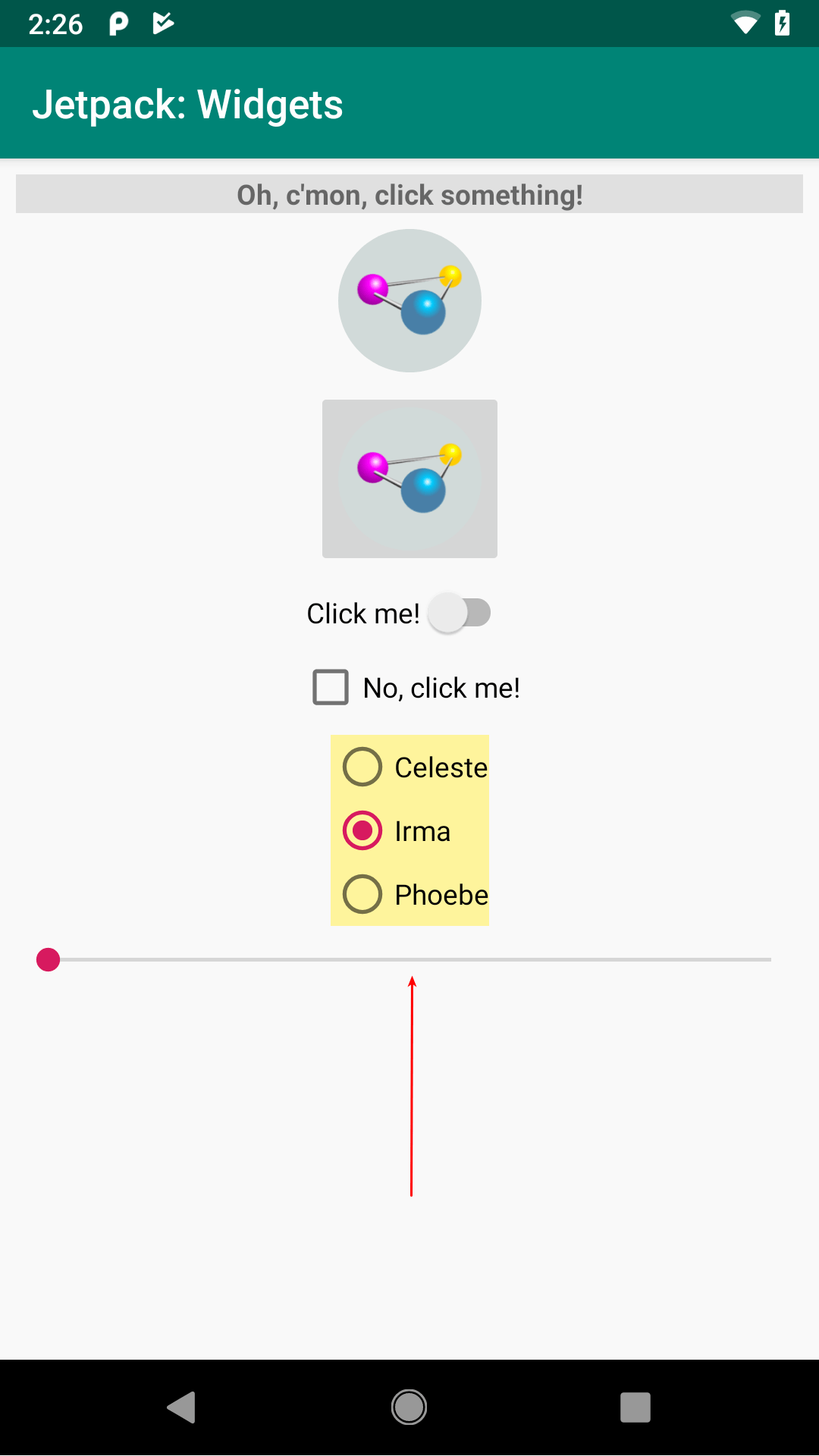
FormWidgets
Sample, with
SeekBar
Highlighted
SeekBar
does not inherit from TextView
, so it has no caption. Most likely, you will want to use an adjacent TextView
to label the SeekBar
, so that the user has an idea of what this value represents.
Android Studio Graphical Layout Editor
The SeekBar
widget can be found in the “Widgets” portion of the Palette in the Android Studio Graphical Layout editor:
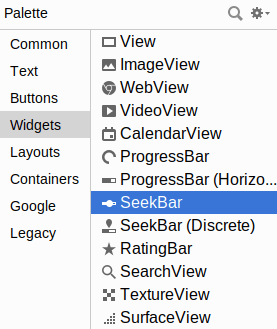
SeekBar
Highlighted
You will notice that there is also an entry for “SeekBar (Discrete)”, where a “discrete” SeekBar
shows tick marks for the values in the range. This can be useful if the range is relatively short (e.g., 0-5). To create a discrete SeekBar
, you need to supply a drawable resource that provides the “tick mark”, showing the user where the thumb will snap to. If you drag a “SeekBar (Discrete)” into your layout resource, you get a SeekBar
with an attribute of style="@style/Widget.AppCompat.SeekBar.Discrete"
. This sets a particular “style” on the SeekBar
, and this style sets the tick mark drawable. We will explore styles in much greater detail later in the book.
Reacting to Events
SeekBar
has setOnSeekBarChangeListener()
, which tells you about changes in the SeekBar
value. However, it also requires you to override a couple of other methods, and so it is a bit more complicated to use than are the other listeners that we have seen in this chapter. We will examine the SeekBar.OnSeekBarChangeListener
more later in this chapter and see how it works.
Prev Table of Contents Next
This book is licensed under the Creative Commons Attribution-ShareAlike 4.0 International license.