ScrollView
: Making It All Fit
Android devices come in all sizes. Some have very large screens, while others have very small screens. The problem with very small screens is that your forms do not always fit the available space, particularly in landscape mode:
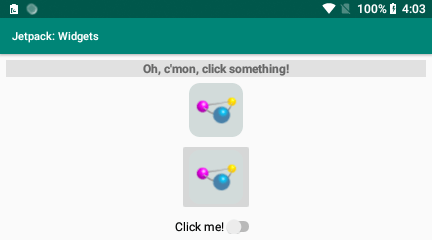
FormWidgets
Sample, In Landscape on a Unihertz Atom
That is why the entire ConstraintLayout
is wrapped in a ScrollView
. ScrollView
provides a vertically-scrolling area for your form. The user can swipe up and down to pan around the form and see all of it.
ScrollView
itself is a very simple container class, holding exactly one child (in this case, the ConstraintLayout
), and making it scrollable. So, our entire activity_main
layout is:
<?xml version="1.0" encoding="utf-8"?>
<ScrollView xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="@dimen/container_padding">
<androidx.constraintlayout.widget.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:id="@+id/log"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:background="@color/log_background"
android:gravity="center_horizontal"
android:text="@string/log_default"
android:textStyle="bold"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ImageView
android:id="@+id/icon"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="@dimen/margin_row"
android:contentDescription="@string/icon_caption"
android:src="@mipmap/ic_launcher"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@id/log" />
<ImageButton
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="@dimen/margin_row"
android:contentDescription="@string/button_caption"
android:src="@mipmap/ic_launcher"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@id/icon" />
<Switch
android:id="@+id/swytch"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="@dimen/margin_row"
android:text="@string/switch_caption"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@id/button" />
<CheckBox
android:id="@+id/checkbox"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="@dimen/margin_row"
android:text="@string/checkbox_caption"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@id/swytch" />
<RadioGroup
android:id="@+id/radioGroup"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="@dimen/margin_row"
android:background="@color/radiogroup_background"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@id/checkbox">
<RadioButton
android:id="@+id/radioButton1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/radiobutton1_caption" />
<RadioButton
android:id="@+id/radioButton2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:checked="true"
android:text="@string/radiobutton2_caption" />
<RadioButton
android:id="@+id/radioButton3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/radiobutton3_caption" />
</RadioGroup>
<SeekBar
android:id="@+id/seekbar"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginTop="@dimen/margin_row"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@id/radioGroup" />
</androidx.constraintlayout.widget.ConstraintLayout>
</ScrollView>
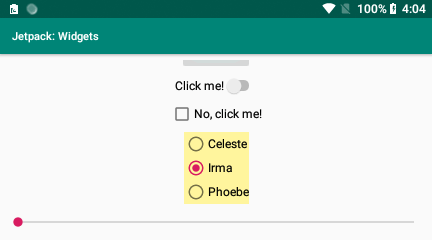
FormWidgets
Sample, In Landscape on a Unihertz Atom, Scrolled Down
Note that there is also a HorizontalScrollView
that will allow your form to be wider than the screen, whereas ScrollView
allows your form to be taller than the screen.
Prev Table of Contents Next
This book is licensed under the Creative Commons Attribution-ShareAlike 4.0 International license.