Collecting a Preference
If there are aspects of your app that are user-configurable, you have two main options for allowing the user to configure them:
- Integrate that configuration into your own UI
- Set up a
PreferenceScreen
A PreferenceScreen
is a way to declare what sorts of configuration options your app has. The PreferenceScreen
can then be used to generate a UI that resembles the Settings app and lets the user configure the items. That generated UI also handles storing the values in a SharedPreferences
object, so you can use the values from within your app code.
In this tutorial, we will set up a PreferenceScreen
, right now to collect just a single preference.
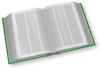
SharedPreferences
and the preference UI system in the "Using Preferences" chapter of Elements of Android Jetpack!
This is a continuation of the work we did in the previous tutorial. The book’s GitLab repository contains the results of the previous tutorial as well as the results of completing the work in this tutorial.
Step #1: Adding a Dependency
There are framework classes related to preferences. However, most of them are deprecated, with replacements in the Jetpack components. To pull in those replacements, we need to add another dependency.
Add this line to the dependencies
closure of your app/build.gradle
file:
implementation "androidx.preference:preference-ktx:1.1.1"
This pulls in the preference-ktx
library, which will give us the Jetpack preference classes, along with some Kotlin extension functions related to preferences.
At this point, the dependencies
closure should resemble:
dependencies {
implementation 'androidx.core:core-ktx:1.6.0'
implementation 'androidx.appcompat:appcompat:1.3.1'
implementation 'androidx.constraintlayout:constraintlayout:2.1.0'
implementation "androidx.recyclerview:recyclerview:1.2.1"
implementation "androidx.navigation:navigation-fragment-ktx:$nav_version"
implementation "androidx.navigation:navigation-ui-ktx:$nav_version"
implementation "androidx.preference:preference-ktx:1.1.1"
implementation 'com.google.android.material:material:1.4.0'
implementation "io.insert-koin:koin-android:$koin_version"
implementation "com.github.jknack:handlebars:4.1.2"
implementation "androidx.room:room-runtime:$room_version"
implementation "androidx.room:room-ktx:$room_version"
kapt "androidx.room:room-compiler:$room_version"
coreLibraryDesugaring 'com.android.tools:desugar_jdk_libs:1.1.5'
testImplementation 'junit:junit:4.13.2'
testImplementation "org.mockito:mockito-inline:3.12.1"
testImplementation "com.nhaarman.mockitokotlin2:mockito-kotlin:2.2.0"
testImplementation 'org.jetbrains.kotlinx:kotlinx-coroutines-test:1.5.1'
androidTestImplementation 'androidx.test.ext:junit:1.1.3'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.4.0'
androidTestImplementation "androidx.arch.core:core-testing:2.1.0"
androidTestImplementation 'org.jetbrains.kotlinx:kotlinx-coroutines-test:1.5.1'
}
This may look like a lot of libraries for a fairly trivial app. However, on the whole, Android app development has become very focused on libraries, and a production-grade app may have a lot more libraries than does this app.
Prev Table of Contents Next
This book is licensed under the Creative Commons Attribution-ShareAlike 4.0 International license.