Getting a Room (And Some Coroutines)
So far, we have been content to have our to-do items vanish when we re-run our app. This was simple and easy to write. However, it is not realistic. Users will expect their to-do items to remain until deleted. To do that, we need our items to survive process termination, and that requires that we save those items somewhere, such as on disk.
In this tutorial, we will start in on that work, setting up database support using Room, a Google-supplied framework that layers atop Android’s native SQLite support. SQLite is a relational database. Through Room, we will create a database containing a table for our to-do items.
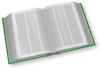
In truth, this app is trivial enough that you could use something simpler for storage, such as storing the items in a JSON file. The bigger the app, the more likely it is that SQLite and Room will be better options for you.
However, even trivial database I/O takes some time, so we want to move that work to background threads. To do that, we will use Kotlin coroutines. Coroutines are a relatively new addition to Kotlin. They try to make it easy for you to write code that looks like it is happening all on one thread, statement after statement, when in reality multiple threads are involved.
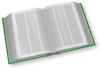
This is a continuation of the work we did in the previous tutorial. The book’s GitLab repository contains the results of the previous tutorial as well as the results of completing the work in this tutorial.
Step #1: Requesting More Dependencies
Room has its own set of dependencies that we need to add to the dependencies
closure in app/build.gradle
.
Room has its own series of versions, independent of anything else that we have used. So, let’s define another version constant in our top-level build.gradle
file. Add this line to the ext
closure:
room_version = "2.3.0"
Then, in app/build.gradle
, add three new dependencies that reference that version constant… and one other dependency:
implementation "androidx.room:room-runtime:$room_version"
implementation "androidx.room:room-ktx:$room_version"
kapt "androidx.room:room-compiler:$room_version"
Room is based heavily on the use of Java annotations, and the androidx.room:room-compiler
dependency will handle those annotations for us at compile time. The androidx.room:room-runtime
dependency is for core Room functionality, while the androidx.room:room-ktx
dependency adds support for Room doing database I/O using coroutines (along with a few other Kotlin extension functions).
The kapt
directive that we are using for room-compiler
says that this dependency contains an annotation processor. That, in turn, requires a new plugin. So, add this line to the other apply plugin
statements towards the top of app/build.gradle
:
id 'kotlin-kapt'
After adding these lines, go ahead and allow Android Studio to sync the project with the Gradle build files.
Prev Table of Contents Next
This book is licensed under the Creative Commons Attribution-ShareAlike 4.0 International license.