Step #10: Adding Navigation Arguments
Before we can display our ToDoModel
, we need to get it in DisplayFragment
. And before we can do that, we need DisplayFragment
to know what model that is.
This gets back to the gap we saw several steps ago, where our display()
function in RosterListFragment
was not doing anything with the ToDoModel
that the user clicked on. We need to use that somehow.
And for that, we will add an argument to our navigation graph.
Open res/navigation/nav_graph.xml
and, in the graphical editor, click on the displayFragment
. In the “Attributes” pane, you will see an “Arguments” section with a + icon:
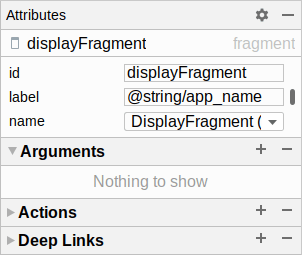
displayFragment
Attributes
Click that + icon in the “Arguments” to open up a dialog to define an argument:
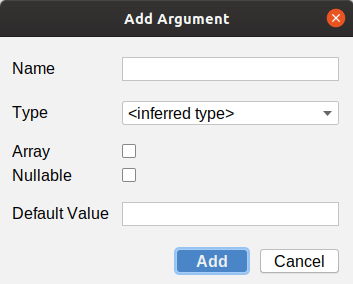
Fill it in as follows:
Property | Value |
---|---|
Name | modelId |
Type | String |
Array | unchecked |
Nullable | unchecked |
Default Value | (leave empty) |
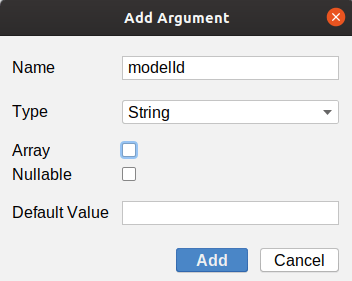
Click “Add” to add it to the navigation graph.
From the Android Studio main menu, choose “Build” > “Make Module ‘app’”. After a few moments, you should get a compile error, from our display()
function in RosterListFragment
:
private fun display(model: ToDoModel) {
findNavController().navigate(RosterListFragmentDirections.displayModel())
}
We added an argument to displayFragment
. Now our action that will navigate to displayFragment
needs a value for that argument, so the RosterListFragmentDirections.displayModel()
function needs our modelId
value. So, modify the function to look like:
private fun display(model: ToDoModel) {
findNavController()
.navigate(RosterListFragmentDirections.displayModel(model.id))
}
Then, over in DisplayFragment
, add this property:
private val args: DisplayFragmentArgs by navArgs()
DisplayFragmentArgs
is code-generated by the Safe Args plugin for the Navigation component. It looks at our declared arguments and creates a Kotlin class that represents them. Moreover, we get a navArgs()
delegate that will build that DisplayFragmentArgs
for us when we first access the args
property. We will be able to use this to access our modelId
value.
Prev Table of Contents Next
This book is licensed under the Creative Commons Attribution-ShareAlike 4.0 International license.