“All Files Access”
Android 11 offers an “All Files Access” capability. The idea is that if your app requests the MANAGE_EXTERNAL_STORAGE
permission, and the user grants it, that you would have unfettered access to most of external and removable storage. There are a few caveats:
-
MANAGE_EXTERNAL_STORAGE
is not adangerous
permission. Instead, it is one of those specialized permissions, likeSYSTEM_ALERT_WINDOW
, where the user needs to go into “Special app access” area of the Settings app to grant the permission. - You still do not have access to
Android/data/
on external storage or any removable volume. This limits the utility ofMANAGE_EXTERNAL_STORAGE
, particularly for backup/restore apps. - Google hints that this permission will be restricted on the Play Store. The result probably will be akin to select other permissions, where you have to fill out a form and get explicit approval to use this permission. Otherwise, your app might be banned from the Play Store. It might get banned anyway, due to a bot, as Google’s policy violation detection bots seem to be unreliable, to the detriment of too many app developers.
- Google has indicated that apps cannot request this permission until 2021, presumably because they do not have the policy violation detection bots fully set up yet.
In the RawPaths
sample module, the activity has a toolbar button with the infinity symbol:
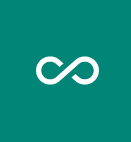
On Android 11 devices, tapping that will request the MANAGE_EXTERNAL_STORAGE
permission, by means of starting an ACTION_MANAGE_APP_ALL_FILES_ACCESS_PERMISSION
activity. This takes a Uri
pointing to your application, using the package:
scheme:
if (item.itemId == R.id.allAccess) {
if (Build.VERSION.SDK_INT >= 30) {
if (hasAllFilesPermission()) {
Toast.makeText(this, R.string.already_permission, Toast.LENGTH_LONG)
.show()
}
val uri = Uri.parse("package:${BuildConfig.APPLICATION_ID}")
startActivity(
Intent(
Settings.ACTION_MANAGE_APP_ALL_FILES_ACCESS_PERMISSION,
uri
)
)
} else {
Toast.makeText(this, R.string.sorry, Toast.LENGTH_LONG).show()
}
This will bring up a Settings screen for the user to grant you the permission:
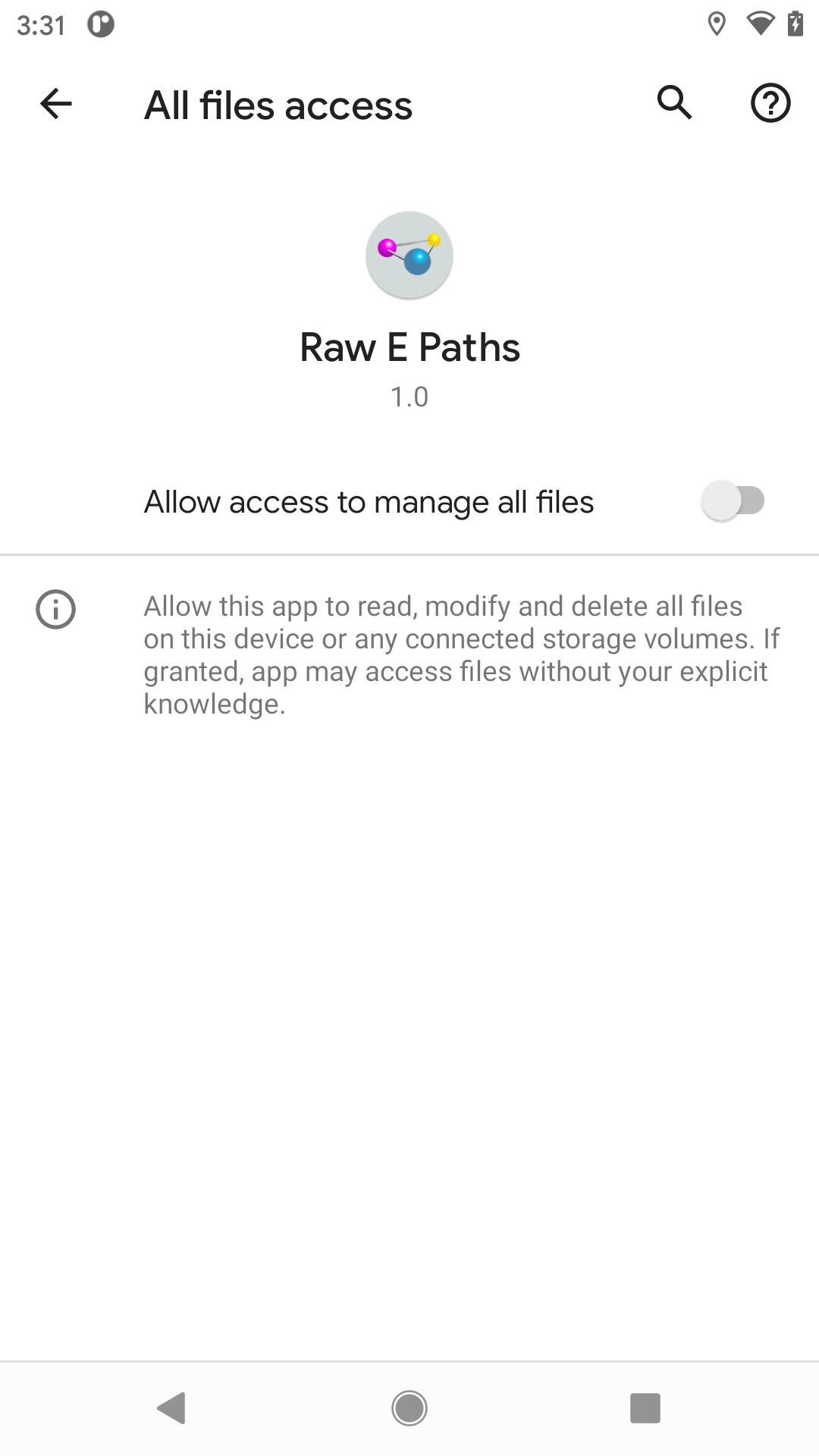
If the user grants you that permission, you now have write access to external storage, akin to what you would have with WRITE_EXTERNAL_STORAGE
on Android 9 and below. In the RawPaths
app, if you have write permission, tapping on a file will make a copy of that file in the same directory, reloading the list to show you the copy. Without MANAGE_EXTERNAL_STORAGE
, you cannot write into many directories (though, as noted earlier, you do have write access to some locations). With MANAGE_EXTERNAL_STORAGE
, you can work with a lot more.
So, on the plus side, MANAGE_EXTERNAL_STORAGE
means it is conceivable that you could have write access, at least to external storage, on Android 11. However, that does not help for Android 10. So, you will still need some other solution for your Android 10 users, as there will be more of them than Android 11 users for a few years.
Detecting This Permission
In Android 11, Environment
now has a pair of isExternalStorageManager()
methods that will tell you if you hold MANAGE_EXTERNAL_STORAGE
.
In theory, the zero-parameter isExternalStorageManager()
would tell you if you can manage external storage, and the one-parameter isExternalStorageManager()
would tell you if you can manage the storage volume containing the supplied File
. In practice, the user seems to only be able to grant MANAGE_EXTERNAL_STORAGE
device-wide, so it is unclear if there is a practical difference between the two.
Prev Table of Contents Next
This book is licensed under the Creative Commons Attribution-ShareAlike 4.0 International license.