Coroutines and ViewModel
Most of the Kotlin samples in this book that need to do background processing will do so using coroutines. We will have suspend
functions that either call other suspend
functions or do work in a designated thread or thread pool:
suspend fun doSomethingCool(): AwesomeMix = withContext(Dispatchers.IO) {
// TODO something that returns an AwesomeMix
}
A suspend
function needs to be called inside of a CoroutineScope
. While Kotlin offers a GlobalScope
that can be used for anything, ideally you use a scope that is more closely tied to the work that is being done.
In Android UI development, that frequently will be a CoroutineScope
associated with a ViewModel
. There is a viewModelScope
extension property available to ViewModel
that provides a CoroutineScope
tied to the lifetime of the ViewModel
. In particular, if the ViewModel
is no longer being used (i.e., it is being called with onCleared()
), the viewModelScope
is also cleaned up.
So, you wind up with code like this:
fun doSomethingCoolAndGetTheResultsOnTheMainApplicationThread() {
viewModelScope.launch(Dispatchers.Main) {
val mix = repo.doSomethingCool()
// TODO something with mix on the main application thread
}
}
(where repo
is an object of the class that has the aforementioned doSomethingCool()
function)
Dispatchers.Main
will mean that while doSomethingCool()
will run on a background thread, we will get the result delivered to us on the main application thread.
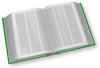
Some of the Kotlin examples later in the book will demonstrate using coroutines.
Prev Table of Contents Next
This book is licensed under the Creative Commons Attribution-ShareAlike 4.0 International license.