Key Elements of Jetpack
Not everything in the Jetpack is of equal importance. This book focuses on those elements that are commonplace for most ordinary Android apps or are tied closely to other commonplace Android app development steps.
AppCompat
As was mentioned earlier in this chapter, Android is over 10 years old. Android changes with every release.
However, Android devices do not get very many OS updates from their manufacturer. Even the best might only get updates for 2-3 years, and there are plenty that never get an update. As a result, Android users use a wide range of Android OS versions. While the changes from release to release may be small or may be large, the combined changes from older versions of Android to newer ones can be vast.
AppCompat tries to help. It gives us an API for our activities and fragments that resembles the latest-and-greatest version of Android. When your app runs on older devices, AppCompat tries to fill in the gaps of UI functionality where it can. While using AppCompat makes your APK a lot larger and makes app development more confusing, many developers are grateful for the backwards-compatibility that it offers.
We will dive more deeply into what AppCompat is, and its relationship to your app, in an upcoming chapter.
Fragment
A layout does not exist on its own. Something has to arrange to use a layout resource and show it on the screen. We saw in the previous chapters that an Activity
can be used for that, and we will start there for seeing how to build Android user interfaces.
However, in many cases, you will wind up using fragments for loading and displaying your layout resources. Fragments are wrappers around layout resources and their widgets. Many apps might consist of a single activity, with each different “screen” being represented by a fragment that uses associated layout resources and code. The user, as part of using the app, will switch from fragment to fragment as needed — for example, clicking a button might cause another fragment to be shown on the screen.
We will look at fragments a bit later in the book.
Navigation
While some apps might have just one screen of information, most have more than one. Users click on widgets and are taken to other screens. Users then press the BACK button and return to the screen they had been on originally. And so forth.
We have had ways of accomplishing this sort of navigation since Android’s introduction. However, it involves Java/Kotlin code, and sometimes quite a bit of it, for complex interactions. Google keeps trying to move some of that sort of work into resources, where it can.
The Navigation library provides a way to declare how to move from screen to screen via resources, with a new editor in Android Studio to assist in defining those resources and depicting your navigation flow.
We will explore this Navigation library as part of our discussion of fragments.
Lifecycles, ViewModel, and LiveData
Most Android devices are phones or tablets. These can be held in portrait or landscape. Well-written apps appear to seamlessly transition between the two as the user rotates the screen.
In reality, screen rotations and other types of “configuration changes” are among the most annoying aspects of Android app development. It turns out that our activities and fragments have “lifecycles”, and they come and go not only based on user navigation but also these configuration changes. Keeping our state as the user rotates the screen is important, as users get irritated if we lose stuff.
To that end, Google nowadays offers two Jetpack pieces to help with this:
- Special code for dealing with lifecycles
- A
ViewModel
class that helps maintain our state across configuration changes
For some developers, configuration changes are the most annoying thing in Android app development. For others, it is threads. We need to do work on background threads to keep our UI “responsive”, accepting and processing user input while that background work is ongoing. This is a pain to manage in its own right, and configuration changes make it that much more difficult. LiveData
objects — held by our ViewModel
— make it somewhat easier to do work in background threads while not losing track of that work if the user rotates the screen, for example.
In this book, we will explore ViewModel
and lifecycle handlers as well as LiveData
and threads.
Room
Many apps need a database on the device. Sometimes, that is the “system of record”, as the data is only on the device. Sometimes, the database serves as more of a local cache, for data that we obtained from a server or for data that we need to get to a server.
Android has had a relational database, called SQLite, in its SDK since the beginning. However, the standard Android APIs for working with this database are a bit crude by modern standards. To that end, Google has created Room, a lightweight object wrapper around SQLite databases, to simplify your database I/O. Room also works nicely with LiveData
, to help you do your database I/O on background threads.
We will explore the basics of Room much later in the book.
WorkManager
Many times, the work that we need to do on background threads has to be performed in near-real time. But sometimes the work that we need to do can happen totally independently from what the user may (or may not) be doing in our UI. So while a “pull to refresh” UI operation requires us to do a refresh right now, a periodic refresh from the server might happen every few hours, even if the user is not in the app right now.
While there have been many solutions over the years for this problem, Google is steering us towards WorkManager
right now. We can teach WorkManager
about background work to be performed, then schedule that work to occur. That schedule might be based in part on time (“do it soon”, “do it every few hours”, etc.). That schedule might be based in part on the state of the device (“do it when the device is on a charger”, “do it when the device is on WiFi”, etc.). Then, WorkManager
will arrange to do the work, even if the user leaves our UI.
We will examine WorkManager
towards the end of the book.
Android KTX
The Android SDK is based on Java, and it has a fairly typical Java API, albeit one with a variety of patterns, based in part on the age of the code.
Kotlin is Google’s recommended language for Android developers going forward, with Java being relegated to the SDK itself. This is similar to how Apple steers developers towards Swift, with Objective-C being used only sporadically.
One of Kotlin’s features is the “extension function”. This is a way for a library to add functions (Java methods) to somebody else’s Java/Kotlin class. For developers using the class, the extension functions are seamlessly integrated with the functions that were part of the class from the outset.
Android KTX is a collection of such extension functions, designed to make common aspects of the Android SDK a bit easier to use.
We will see Android KTX code sprinkled throughout this book.
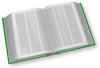
Testing
No software project is complete without testing. We have already seen how Android projects can have instrumented tests and unit tests. We are given stub tests when we create our project, just to get us going, but we will need to write a lot more tests before we are done.
We will be exploring testing more later in the book.
Compose
The most recent major addition to the Jetpack is Jetpack Compose.
Jetpack Compose is a new way of implementing user interfaces in Android. In effect, it is a Kotlin domain-specific language (DSL) for implementing user interfaces. It replaces things like layout resources with pure Kotlin code. In the coming years, it is likely that Compose will become a very important part of the Android development ecosystem.
However, Compose only received a stable 1.0.0 release in July 2021. While it can be used for shipping apps, it does have a fair number of bugs which may impede your progress. In addition, only a tiny fraction of the world’s Android UIs are created using Compose today, and only a tiny fraction of the world’s knowledge of Android app development centers around Compose.
This book does not cover Compose. You will want to keep an eye on how rapidly Compose gets adopted by developers and make plans to learn Compose at the appropriate time.
Prev Table of Contents Next
This book is licensed under the Creative Commons Attribution-ShareAlike 4.0 International license.