Accessing the Internet
Nearly every Android device has Internet access. It is fairly likely that you will have interest in accessing the Internet from your Android app.
Android has a lot to offer here, both in terms of what is part of the OS and in terms of the wide range of add-on libraries to assist in Internet access.
This chapter covers some general Internet access options for Android apps, then walks through a sample using two popular libraries: Retrofit and Glide.
NOTE: This chapter will use “the Internet” to refer to any networking. The rules and options outlined here are not solely for accessing the public Internet but also for accessing private networks, such as an office network.
An API Roundup
There are lots and lots of ways that your app can access the Internet. Some APIs are part of the Android SDK, while some are third-party libraries layered atop the SDK. Here are some of the more popular and better-known options.
Socket
In the end, anything in Java/Kotlin code that is working with the Internet will wind up using a Socket
. If you are trying to create a library that implements some obscure or new Internet protocol, you might find yourself using Socket
to connect to some server, then implementing all of the communications yourself.
Most developers should not need to do this. Most major protocols have implementations available for Android, whether in the OS or in a library. All else being equal, you are better served using an existing implementation rather than “rolling your own”.
HttpUrlConnection
The original HTTP API in Java centers around HttpUrlConnection
and related classes. Android supports this, and it is the only supported direct HTTP API in the Android SDK at the present time. However, the HttpUrlConnection
API is very old, and there are better alternatives, such as OkHttp. In fact, Android’s HttpUrlConnection
is built on top of a forked copy of OkHttp.
WebView
If your objective is to display Web content to the user, most likely you will want to use WebView
. This is a widget in the Android SDK that renders Web content, much like a browser. You can put a WebView
in your activities or fragments and hand the WebView
the content to display, in the form of HTML strings or URLs.
Conversely, if you are looking to talk to a Web service or otherwise engage in HTTPS communications without displaying HTML/CSS/JavaScript to the user, you will want to use something other than WebView
.
DownloadManager
If your objective is to download a file from a publicly-accessible URL, you could use DownloadManager
. This too is part of the Android SDK, and its job is to perform this sort of download. It handles some of the complexity for you, such as dealing with network disconnections and picking up the download later from where it left off.
However, DownloadManager
has many limitations. For example, you have no means of providing a session cookie or authentication header to the Web server — the URL must be one that can be used without additional HTTP stuff. You have limited places for storing the downloaded result. By default, the user will see a notification in their status bar, indicating the progress of the download. And so on.
For its narrow purpose, DownloadManager
may be OK, but is not designed for working with arbitrary Web services and does not offer much flexibility.
Volley
Google offers its own add-on library for Internet access, called Volley. Volley offers a cleaner API for accessing Web services and downloading images than you get with HttpUrlConnection
, even though “under the covers” Volley uses HttpUrlConnection
to do its work. Beyond that, Volley’s primary “claims to fame” are:
- It was created by Google
- It reportedly is used by the Play Store and some other Google apps
As such, some developers prefer to use this, over other options, as it is easier to get the library approved by decision-makers.
OkHttp
Perhaps the most popular option for generic HTTP requests is OkHttp. Published by Square, OkHttp is frequently updated and has good developer and community support. OkHttp is a first-class HTTP client, working directly with Socket
(as opposed to being layered atop HttpUrlConnection
). OkHttp has a clean yet rich API for making and monitoring requests and responses.
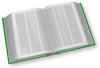
OkHttp also serves as the “plumbing” for various other libraries, including most of those listed below.
Retrofit
Square’s Retrofit is a library layered atop of OkHttp that aims to simplify making REST-style Web service calls. You use annotations on a Java/Kotlin interface
to describe the REST URLs that you wish to access, along with the HTTP operations to perform (e.g., GET
, POST
, PUT
). If you combine Retrofit with a parsing library suitable for the Web service — such as a JSON parser for Web services serving JSON responses — Retrofit allows you to craft an API that feels like it is just a local function call yet returns an object tree representing the response.
We will examine the use of Retrofit in the sample app.
Apollo-Android
If your server is using GraphQL instead of REST, Apollo-Android is basically “Retrofit for GraphQL”. You provide your GraphQL in the form of documents in a graphql/
project directory. Apollo-Android plugins will generate Java/Kotlin classes that implement that API and represent the responses. You can then use that API at runtime, with OkHttp handling the HTTPS communications.
Image Loaders
A common need in Android apps is to display images from URLs. There are lots of image-loading libraries that handle that work for you with a simple API. The two most popular are Glide and Picasso. Both can integrate with OkHttp, which is particularly important if you are using OkHttp-based libraries elsewhere and wish to have a common configuration for things like logging, proxy servers, and so on.
We will examine the use of Glide in the sample app.
Specialized APIs
If you are looking to communicate with an existing third-party Web service, there might be dedicated libraries for that purpose. That third party might offer their own SDK, and independent developers may have created other libraries.
In general, if a Web service has an official SDK, you are better off using that. If nothing else, when it comes time to get support, the Web service developers may be expecting you to use their SDK and may have difficulty helping you debug your own manual HTTPS code.
Prev Table of Contents Next
This book is licensed under the Creative Commons Attribution-ShareAlike 4.0 International license.