Step #2: Adjusting Our Theme
The app bar color is one aspect of our app that is managed by a theme. A theme provides overall “look and feel” instructions for our activity, including the app bar color.
Your project already has a custom theme declared. If you look in your res/values/
directory, you will see a styles.xml
file — open that in Android Studio:
<resources>
<!-- Base application theme. -->
<style name="Theme.ToDo" parent="Theme.AppCompat.Light.DarkActionBar">
<!-- Customize your theme here. -->
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="colorAccent">@color/colorAccent</item>
</style>
</resources>
Here, we see that we have a style resource named Theme.ToDo
. Style resources can be applied either to widgets (to tailor that particular widget) or as a theme to an activity or entire application. By convention, style resources with “Theme” in the name are themes. This particular theme inherits from Theme.AppCompat.Light.DarkActionBar
, as indicated in the parent
attribute. And, it associates our three colors with three roles in the theme:
-
colorPrimary
will be the dominant color and will be the background color of the app bar -
colorPrimaryDark
mostly is used for coloring the status bar (the bar at the top of the screen that has the time, battery level, signal strength, etc.) -
colorAccent
will be used for certain pieces of widgets, such as the text-selection cursor inEditText
widgets
However, we will be configuring the app bar ourselves with a Toolbar
. By default, a DarkActionBar
theme will add an app bar for us, which we do not need.
Another consideration is whether the overall color scheme will be “light” or “dark”. Historically, Google would steer developers towards a “light” theme, with dark text on a mostly-white background. This is not great for people using apps in dark places or at night, though. In Android 10, Google is starting to steer developers towards having two themes: a light one for normal use and a “dark mode” one. This means that you, the developer, have four main courses of action:
- Ignore Google and stick with a light theme
- Use two themes
- Use a single “day-night” theme with two sets of colors
- Use a dark theme all the time
The latter approach is simplest, in that it accommodates “dark mode” scenarios and does not require that you deal with two themes or two sets of colors. So, that is what we will use in this tutorial.
With that in mind, replace Theme.AppCompat.Light.DarkActionBar
in res/value/styles.xml
with Theme.AppCompat.NoActionBar
. Removing the Light
portion gives us a dark theme, and replacing DarkActionBar
with NoActionBar
removes the automatically-added app bar.
The resulting resource should look like:
<resources>
<!-- Base application theme. -->
<style name="Theme.ToDo" parent="Theme.AppCompat.NoActionBar">
<!-- Customize your theme here. -->
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="colorAccent">@color/colorAccent</item>
</style>
</resources>
Note that the color swatches in the gutter in this Android Studio are clickable as well, bringing up the same editor as before, this time defaulting to the “Resources” tab:
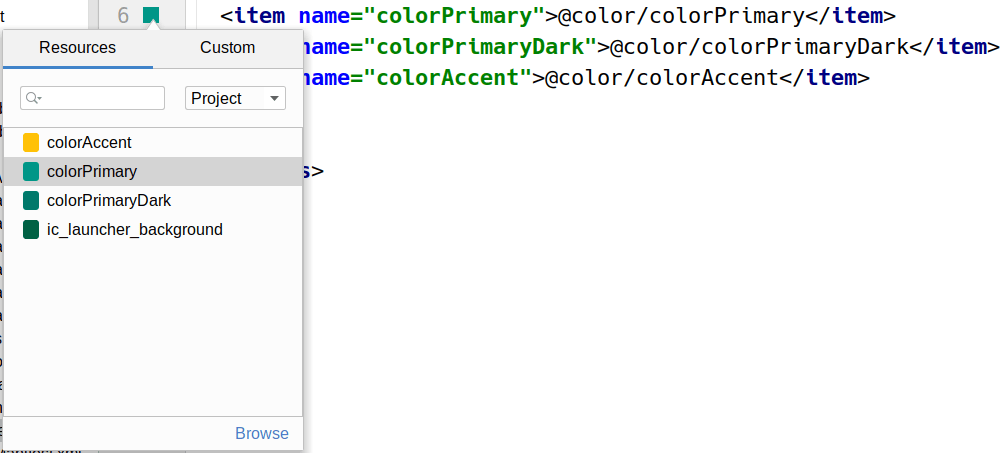
Our AndroidManifest.xml
file already ties in this custom theme, via the android:theme
attribute in the <application>
element:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.commonsware.todo">
<supports-screens
android:largeScreens="true"
android:normalScreens="true"
android:smallScreens="true"
android:xlargeScreens="true"/>
<application
android:allowBackup="false"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.ToDo">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Prev Table of Contents Next
This book is licensed under the Creative Commons Attribution-ShareAlike 4.0 International license.