Step #3: Inflating Our Layout
Right now, this fragment does not do anything, and we need it to display our user interface. So, with your cursor inside the { }
of the class, press Ctrl-O to bring up a list of methods that we could override:
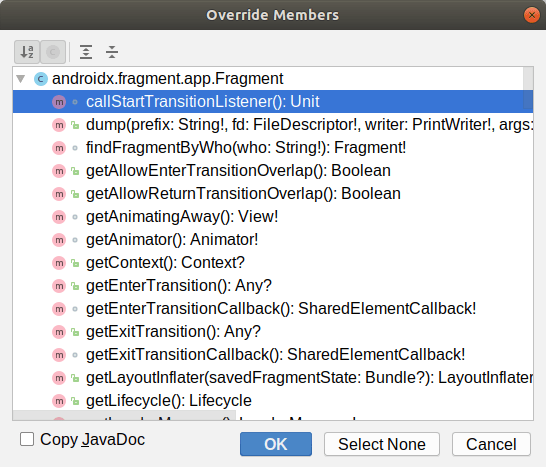
If you start typing with that dialog on the screen, what you type in works as a search mechanism, jumping you to the first method that resembles what you typed in. So, start typing in onCreateView
, until that becomes the selected method:
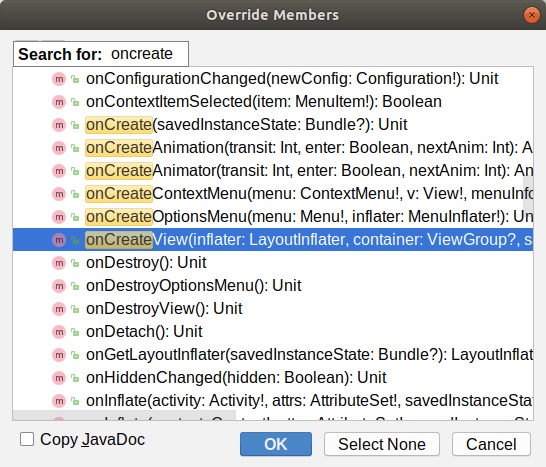
Then, click “OK” to add a stub implementation of that method to your RosterListFragment
:
package com.commonsware.todo
import android.os.Bundle
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import androidx.fragment.app.Fragment
class RosterListFragment : Fragment() {
override fun onCreateView(
inflater: LayoutInflater,
container: ViewGroup?,
savedInstanceState: Bundle?
): View? {
return super.onCreateView(inflater, container, savedInstanceState)
}
}
The override
keyword means that we are overriding an existing function that we are inheriting from Fragment
.
The job of onCreateView()
of a fragment is to set up the UI for that fragment. In MainActivity
, right now, we are doing that by calling setContentView(R.layout.activity_main)
. We want to use that layout file here instead. To do that, modify onCreateView()
to look like:
package com.commonsware.todo
import android.os.Bundle
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import androidx.fragment.app.Fragment
class RosterListFragment : Fragment() {
override fun onCreateView(
inflater: LayoutInflater,
container: ViewGroup?,
savedInstanceState: Bundle?
): View? {
return inflater.inflate(R.layout.todo_roster, container, false)
}
}
Here, we use the supplied LayoutInflater
. To “inflate” in Android means “convert an XML resource into a corresponding tree of Java objects”. LayoutInflater
inflates layout resources, via its family of inflate()
methods. We are specifically saying:
- Inflate
R.layout.todo_roster
- Its widgets will eventually go into the
container
supplied toonCreateView()
- Do not put those widgets in that container right now, as the fragment system will handle that for us at an appropriate time
In practice, you could skip the return type of the function. However, Android Studio will complain about that, as Kotlin cannot tell whether onCreateView()
is allowed to return null
or not. So, to eliminate the Android Studio warning, we have onCreateView()
return View?
specifically.
Note that at this point you cannot see this fragment. We need to take some steps to have MainActivity
display it, which we will handle in the next tutorial.
Prev Table of Contents Next
This book is licensed under the Creative Commons Attribution-ShareAlike 4.0 International license.