Step #7: Fixing the Empty Text
At this point, there are two situations when we have an empty list:
- If there are no to-do items at all
- If there are no to-do items in the current filter mode (e.g., all of the items are outstanding, and the filter mode is set to
COMPLETED
)
This is going to be confusing to the user — the user might not realize that the reason their list is empty is that all of the relevant to-do items have been removed from the list via the filter.
We should improve this.
First, go into res/values/strings.xml
and add a new string resource:
<string name="msg_empty_filtered">Click the + icon to add a todo item, or change your filter to show other items</string>
(note: this will be shown in the book as split across multiple lines, but you are welcome to have it be all on one line in your project, if you wish)
Next, open res/layout/todo_roster.xml
in the IDE. Click on our empty
TextView
. In the “Layout” section of the “Attributes” pane, give the widget 8dp
of margin on all four sides, so it does not run all the way to the edges of the screen:
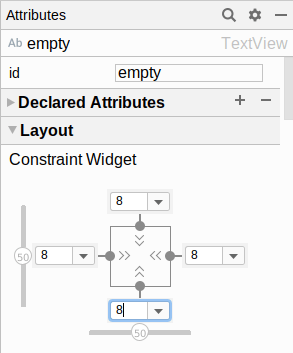
Then, open the “gravity” options:
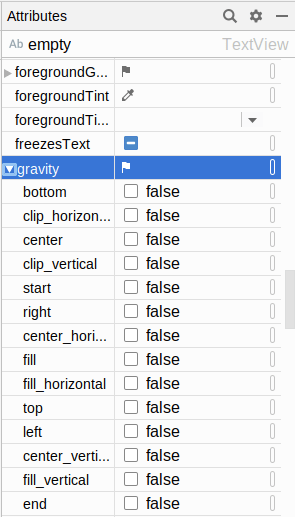
Check the “center” option, which will cause our text to be centered within the space being occupied by the TextView
.
Then, in RosterListFragment
, update the motor.states
observer in onViewCreated()
to be:
viewLifecycleOwner.lifecycleScope.launchWhenStarted {
motor.states.collect { state ->
adapter.submitList(state.items)
binding?.apply {
loading.visibility = View.GONE
when {
state.items.isEmpty() && state.filterMode == FilterMode.ALL -> {
empty.visibility = View.VISIBLE
empty.setText(R.string.msg_empty)
}
state.items.isEmpty() -> {
empty.visibility = View.VISIBLE
empty.setText(R.string.msg_empty_filtered)
}
else -> empty.visibility = View.GONE
}
}
}
}
Here, we use a when
block to handle the three cases:
- Showing the original empty message if we have no items and have a
FilterMode
ofALL
- Showing the new empty message if we have no items and have some other
FilterMode
- Removing the empty message if we have items to show
Now, if you run the app, you will see the empty message centered, and you will see the new empty message if you have items but they are all hidden by the filter:
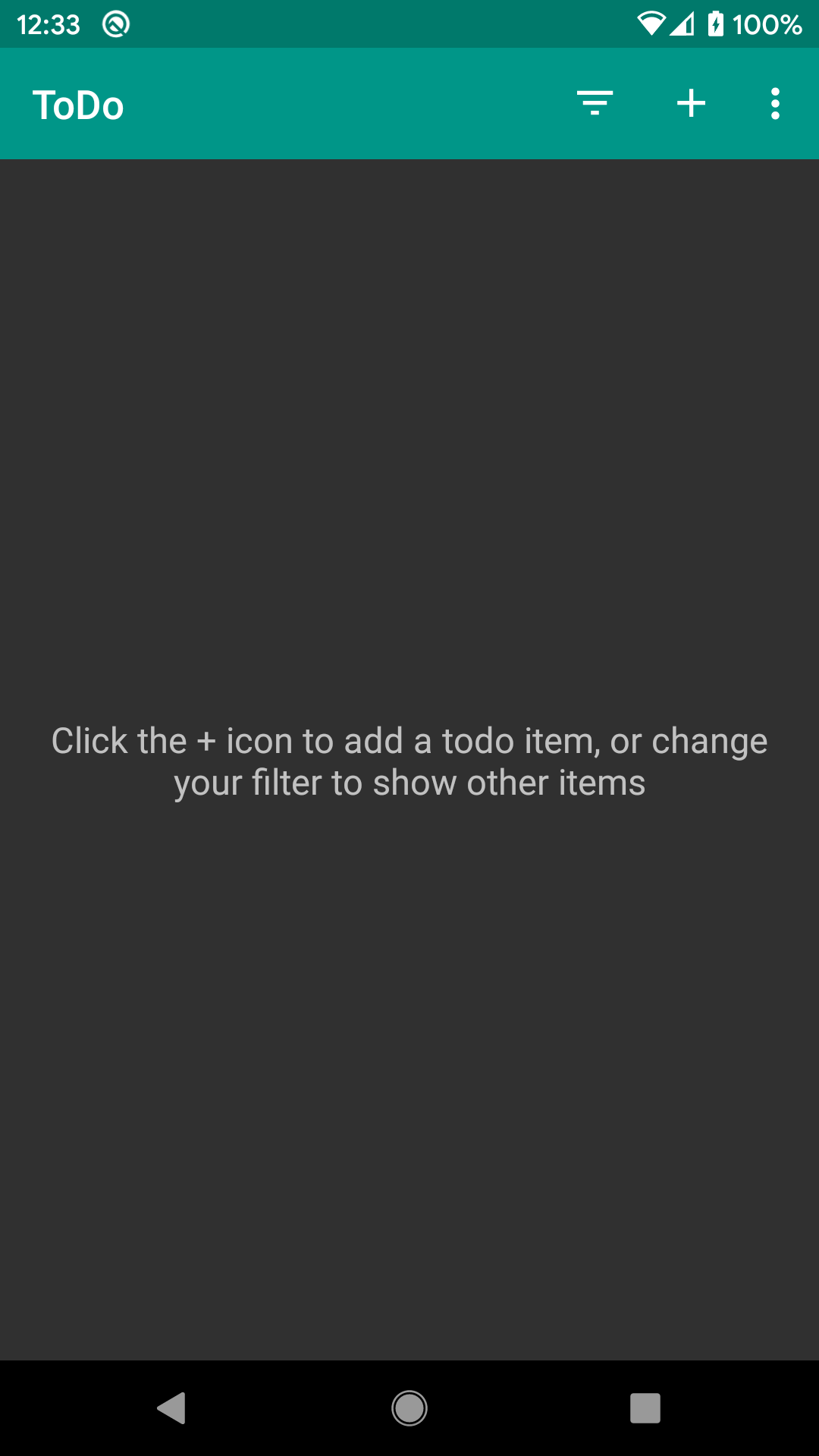
Prev Table of Contents Next
This book is licensed under the Creative Commons Attribution-ShareAlike 4.0 International license.