Testing a Motor
We think that our app works, in that we can see it working when we use the app’s UI. Besides, this is a book, and books never have mistakes, right?
(right?!?)
In the real world, though, you do not have a set of tutorials for every bit of code that you want to write. Along the way, writing tests will help you confirm that the code that you wrote actually works, including for scenarios that are supported by the API that you created but might not be used yet by the UI. So, in this tutorial, we will start adding some tests to our project.
This is a continuation of the work we did in the previous tutorial. The book’s GitLab repository contains the results of the previous tutorial as well as the results of completing the work in this tutorial.
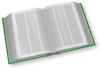
Step #1: Examine Our Existing Tests
The good news is that the project you imported to start these tutorials already has some tests written for you.
(no, this does not mean that you are done with testing)
Tests in Android modules go into “source sets” that are peers of main/
. If you examine your project in Android Studio, you will see that there are three directories in app/src/
: androidTest/
, main/
, and test/
:
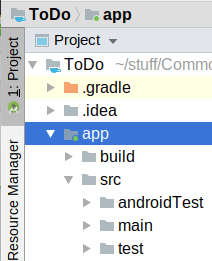
androidTest/
holds “instrumented tests”. Simply put, these are tests of our code that run on an Android device or emulator, just as our app does. If you go into that directory, you will see that it has its own java/
tree, with an ExampleInstrumentedTest
defined there:
package com.commonsware.todo
import androidx.test.platform.app.InstrumentationRegistry
import androidx.test.ext.junit.runners.AndroidJUnit4
import org.junit.Test
import org.junit.runner.RunWith
import org.junit.Assert.*
/**
* Instrumented test, which will execute on an Android device.
*
* See [testing documentation](http://d.android.com/tools/testing).
*/
@RunWith(AndroidJUnit4::class)
class ExampleInstrumentedTest {
@Test
fun useAppContext() {
// Context of the app under test.
val appContext = InstrumentationRegistry.getInstrumentation().targetContext
assertEquals("com.commonsware.todo", appContext.packageName)
}
}
test/
holds “unit tests”. These are tests of our code that run directly on our development machine. On the plus side, they run much faster, as we do not have to copy the test code over to a device or emulator, and a device or emulator is going to be slower than our development machine (usually). On the other hand, our development machine is not running Android, so we cannot easily test code that touches Android-specific classes and methods. Like androidTest/
, test/
has its own java/
tree, with an ExampleUnitTest
defined there:
package com.commonsware.todo
import org.junit.Test
import org.junit.Assert.*
/**
* Example local unit test, which will execute on the development machine (host).
*
* See [testing documentation](http://d.android.com/tools/testing).
*/
class ExampleUnitTest {
@Test
fun addition_isCorrect() {
assertEquals(4, 2 + 2)
}
}
Neither of these test very much, let alone anything related to our own code.
Prev Table of Contents Next
This book is licensed under the Creative Commons Attribution-ShareAlike 4.0 International license.