Step #3: Creating a Stub Adapter
A RecyclerView.ViewHolder
is managed by a RecyclerView.Adapter
. The Adapter
knows how to create instances of ViewHolder
and how to populate them with data as the user views items in the list. So, we need a RecyclerView.Adapter
implementation.
Right-click over the com.commonsware.todo
Java package and choose “New” > “Kotlin File/Class” from the context menu. Fill in RosterAdapter
as the “Name” and choose “Class” from the list of Kotlin structures. Then, press Enter or Return to create a stub Kotlin class.
Then, replace the generated contents with:
package com.commonsware.todo
import androidx.recyclerview.widget.ListAdapter
class RosterAdapter : ListAdapter<ToDoModel, RosterRowHolder>() {
}
Here, we are using a subclass of RecyclerView.Adapter
named ListAdapter
. There are two classes named ListAdapter
in the Android SDK — be sure that you are using androidx.recyclerview.widget.ListAdapter
. ListAdapter
knows how to manage a list of items. In particular, when we replace that list, it knows how to make incremental changes to the RecyclerView
contents to update it to match the new list. ListAdapter
takes two data types:
- The type of model data that will be in the list (
ToDoModel
) - The
RecyclerView.ViewHolder
that will be used for the views (RosterRowHolder
)
The stub RosterAdapter
will show two errors. One is that we are not passing a required constructor parameter to ListAdapter
– we will address that shortly. The other error is that we are missing some functions required by ListAdapter
, as it is an abstract
class.
To address that bug, with the text cursor in the RosterAdapter
name, press Alt-Enter (Option-Return on macOS) and choose “Implement members” from the quick-fix popup menu:
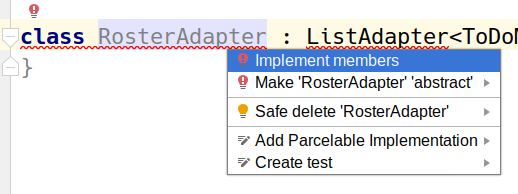
This will pop up a dialog box with functions that you can implement:
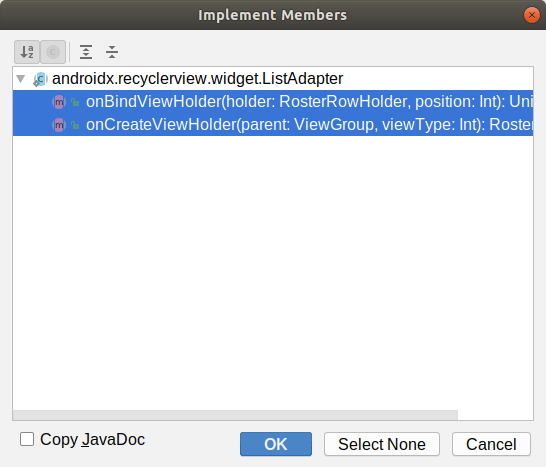
Select both functions in the list, then click “OK”.
That will update the Kotlin code to look something like:
package com.commonsware.todo
import android.view.ViewGroup
import androidx.recyclerview.widget.ListAdapter
class RosterAdapter : ListAdapter<ToDoModel, RosterRowHolder>() {
override fun onCreateViewHolder(
parent: ViewGroup,
viewType: Int
): RosterRowHolder {
TODO("Not yet implemented")
}
override fun onBindViewHolder(holder: RosterRowHolder, position: Int) {
TODO("Not yet implemented")
}
}
onCreateViewHolder()
and onBindViewHolder()
will need real implementations at some point — we will address that later in this tutorial.
Prev Table of Contents Next
This book is licensed under the Creative Commons Attribution-ShareAlike 4.0 International license.